How to Post a Tweet Using Tweepy and Twitter API V2
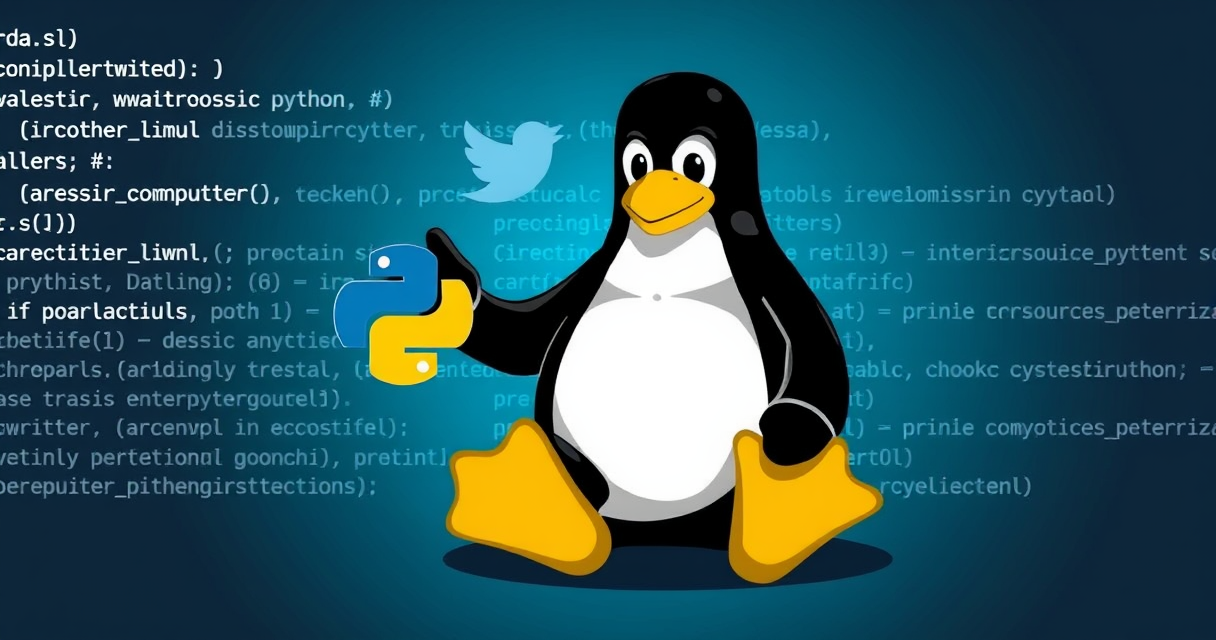
In this blog post, we will walk through the steps to post a tweet using the Tweepy library and Twitter API v2. Tweepy is a popular Python library that provides easy access to the Twitter API. By the end of this tutorial, you will be able to authenticate with Twitter and post a tweet programmatically.
Prerequisites
Before we begin, make sure you have the following:
- Python 3.6+: Ensure you have Python installed on your machine.
- Tweepy Library: Install the Tweepy library using pip.
- Twitter Developer Account: You need to have a Twitter Developer account to get the necessary API credentials.
Step 1: Install Tweepy
First, you need to install the Tweepy library. Open your terminal and run the following command:
pip install tweepy
Step 2: Get Twitter API Credentials
To interact with the Twitter API, you need to create a Twitter Developer account and obtain the following credentials:
- API Key
- API Key Secret
- Access Token
- Access Token Secret
- Bearer Token
You can get these credentials by creating an app in the Twitter Developer Portal.
Step 3: Write the Python Script
Now, let's write the Python script to post a tweet. Replace the placeholder values with your actual Twitter API credentials.
import tweepy
# Replace these values with your own credentials
API_KEY = 'API_KEY'
API_KEY_SECRET = 'API_KEY_SECRET'
ACCESS_TOKEN = 'ACCESS_TOKEN'
ACCESS_TOKEN_SECRET = 'ACCESS_TOKEN_SECRET'
BEARER_TOKEN = 'BEARER_TOKEN'
# Authenticate to Twitter using OAuth 2.0 Bearer Token
client = tweepy.Client(bearer_token=BEARER_TOKEN,
consumer_key=API_KEY,
consumer_secret=API_KEY_SECRET,
access_token=ACCESS_TOKEN,
access_token_secret=ACCESS_TOKEN_SECRET)
# Post a tweet
tweet = "Hello, world! This is a tweet from Tweepy using Twitter API v2."
response = client.create_tweet(text=tweet)
print("Tweet posted successfully!", response)
Step 4: Run the Script
Save the script in a file (e.g., post_tweet.py
) and run it:
If everything is set up correctly, you should see a confirmation message indicating that the tweet was posted successfully.
You can see all the tweets created by my python script in here:
Extra
In this example, I'll demonstrate how to tweet with an image upload using the Tweepy library.
import tweepy
import subprocess
# Replace these values with your own credentials
API_KEY = 'API_KEY'
API_KEY_SECRET = 'API_KEY_SECRET'
ACCESS_TOKEN = 'ACCESS_TOKEN'
ACCESS_TOKEN_SECRET = 'ACCESS_TOKEN_SECRET'
BEARER_TOKEN = 'BEARER_TOKEN'
auth = tweepy.OAuth1UserHandler(API_KEY, API_KEY_SECRET, ACCESS_TOKEN, ACCESS_TOKEN_SECRET)
api = tweepy.API(auth)
# Authenticate to Twitter using OAuth 2.0 Bearer Token
client = tweepy.Client(bearer_token=BEARER_TOKEN,
consumer_key=API_KEY,
consumer_secret=API_KEY_SECRET,
access_token=ACCESS_TOKEN,
access_token_secret=ACCESS_TOKEN_SECRET)
# Upload the image using the API object
media = api.media_upload(image_name="image07.png")
# Post a tweet
tweet = "Test Uploading Image"
response = client.create_tweet(text=tweet, media_ids=[media.media_id])
print("Tweet posted successfully!", response)
Conclusion
In this tutorial, we demonstrated how to post a tweet using the Tweepy library and Twitter API v2. By following these steps, you can easily integrate Twitter functionality into your Python applications. Happy tweeting!
Additional Resources
Feel free to send me a messsage if you have any questions or run into any issues. Happy coding!