Creating CLI Tool with Click in Python Part-2
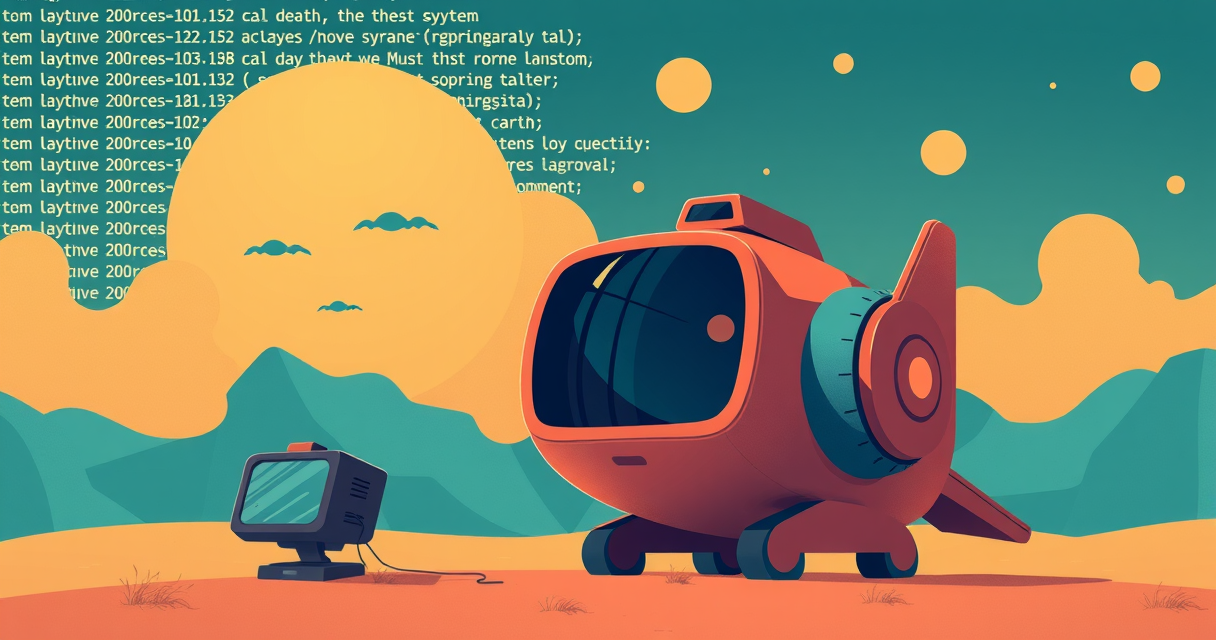
This is the second part of the python click library post. You can view the first part here.
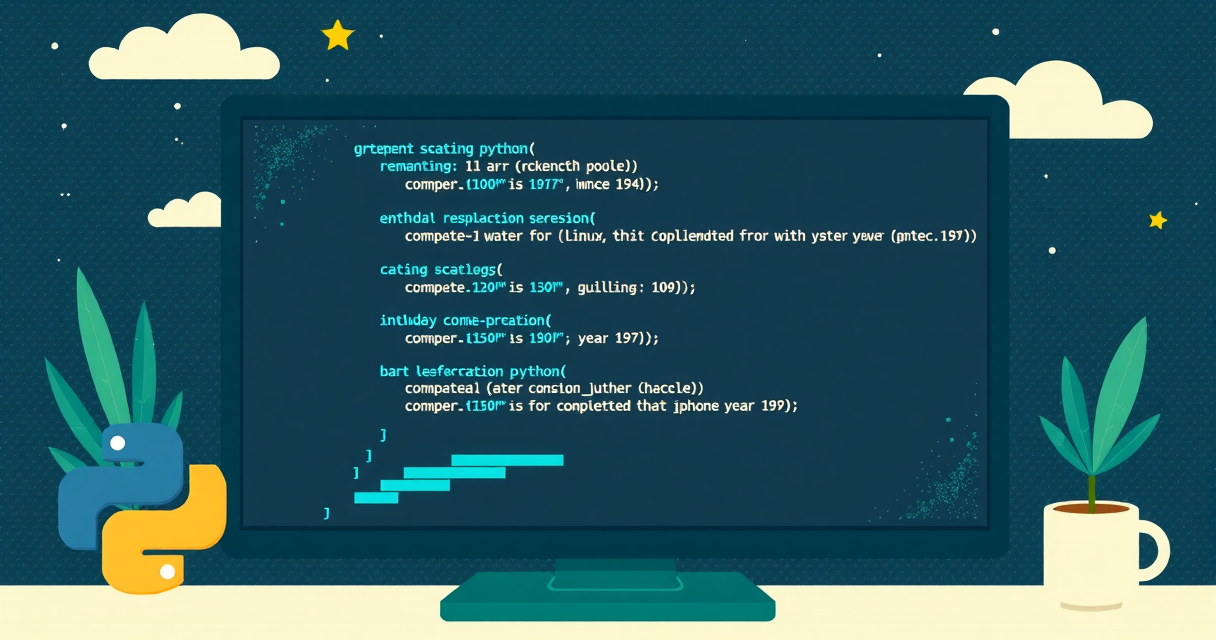
Example: Grouping Commands
Below is a simple example demonstrating how to use the @click.group()
decorator to group multiple commands:
import click
@click.group()
def cli():
pass
@cli.command()
@click.argument('first_name')
def greet(first_name):
click.echo(f"Hello {first_name}!")
@cli.command()
@click.argument('first_name')
def goodbye(first_name):
click.echo(f"Goodbye! {first_name}!")
if __name__ == "__main__":
cli()
Explanation
- @click.group(): This decorator is used to define a group of commands. The
cli()
function serves as the entry point for the group. - @click.command(): This decorator is used to define individual commands. In this example, we have two commands:
greet
andgoodbye
. - @click.argument('first_name'): This decorator is used to define a positional argument for the commands.
By grouping the greet
and goodbye
commands under the cli
group, you can easily manage and invoke them from the command line:
python script.py greet Melih
# Output: Hello Melih!
python script.py goodbye Melih
# Output: Goodbye! Melih!
Importance of click.group
The click.group
functionality is important because it allows you to:
- Organize Commands: Grouping commands makes your CLI application more organized and easier to navigate.
- Modular Code: It helps in keeping the code modular by separating different functionalities into distinct commands.
- Scalability: As your application grows, you can easily add more commands to the group without cluttering the main script.
Conclusion
The click.group
functionality in the Click library provides a structured way to manage multiple commands in a CLI application. Whether you're building a simple tool or a complex application, Click's group functionality helps keep your code organized and scalable.