Convert Text to Speech in Python with Pyttsx3
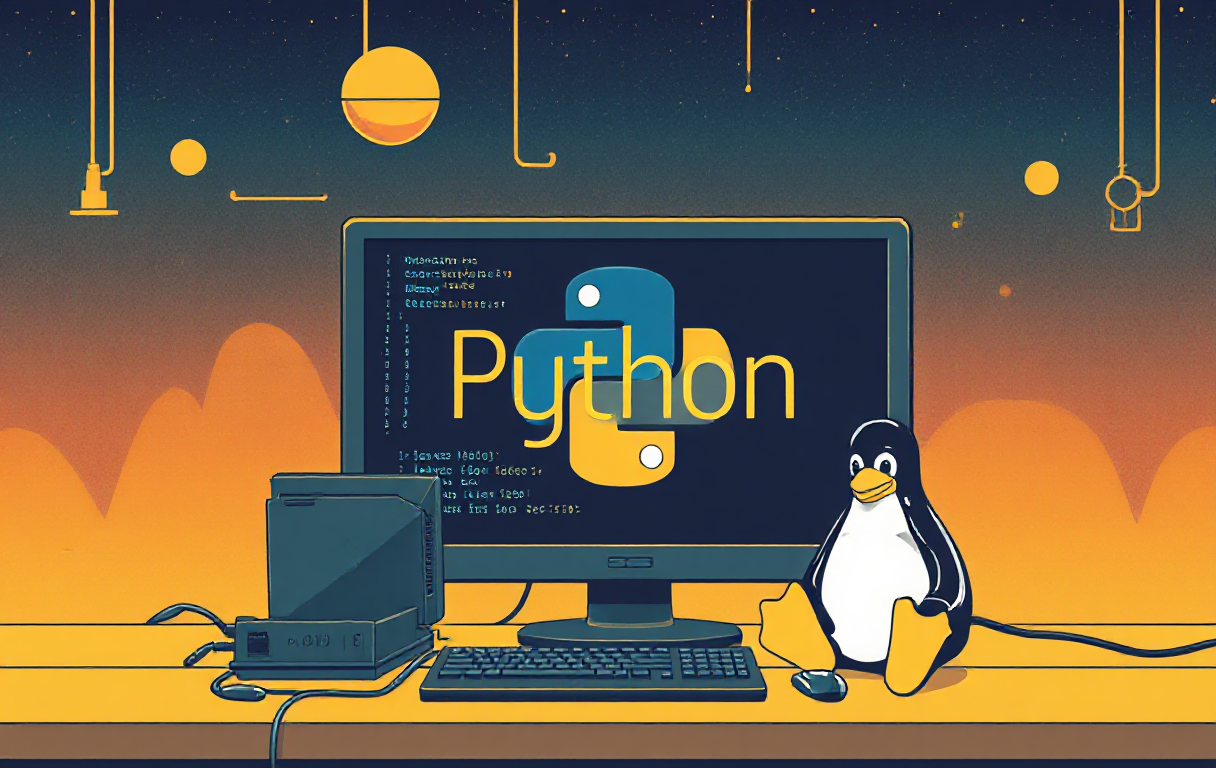
Are you looking to add text-to-speech functionality to your Python projects? In this blog post, I'll show you how to use the Pyttsx3 library to convert text to speech with just a few lines of code. This is a simple yet powerful way to make your applications more accessible and interactive.
What is Pyttsx3?
Pyttsx3 is a text-to-speech conversion library in Python. Unlike other libraries, it works offline and is compatible with both Python 2 and 3. It supports multiple TTS engines, including Sapi5 on Windows and NSSpeechSynthesizer on MacOS.
Getting Started
First, you'll need to install the Pyttsx3 library. You can do this using pip:
pip install pyttsx3
Basic Usage
Here's a simple script that demonstrates how to use Pyttsx3 to convert text to speech:
import pyttsx3
# Initialize the TTS engine
engine = pyttsx3.init()
# Set properties
rate = engine.getProperty('rate') # Get the current speech rate
engine.setProperty('rate', rate - 25) # Decrease the speech rate
# Text to be spoken
text = "Hello, this is a text-to-speech test."
# Speak the text
engine.say(text)
engine.runAndWait()
Explanation
- Initialize the TTS Engine: The
pyttsx3.init()
function initializes the text-to-speech engine. - Set Properties: You can adjust various properties of the speech engine. In this example, we adjust the speech rate to make it slower.
- Speak the Text: The
engine.say(text)
function queues the text to be spoken, andengine.runAndWait()
processes the queue.
Additional Features
Pyttsx3 allows you to customize the voice and other properties. For example, you can change the voice to female by uncommenting and modifying the following lines:
voices = engine.getProperty('voices') # Get the available voices
engine.setProperty('voice', voices[1].id) # Change the voice (0 for male, 1 for female)
Watch the Video Tutorial
For a more detailed walkthrough, check out my youtube video below where I explain each step and demonstrate the code in action.
Source Code
You can find the complete source code for this project on my github account.
Conclusion
Adding text-to-speech functionality to your Python projects is straightforward with the Pyttsx3 library. Whether you're building an accessibility feature or just want to add some interactivity, Pyttsx3 is a great tool to have in your toolkit.
Feel free to contact me if you have any questions or suggestions. Happy coding!